Pythonで添付ファイル付きメールを送信するには?
Pythonには標準でsmtplibライブラリでメール送信する機能が備わっており、簡単にメールを送信することが可能となっています。
- 自動化の促進:
smtplib
を使用すると、定期的なレポートの送信、アラートの通知、確認メールの送信など、多くの業務プロセスを自動化できます。これにより、人的ミスを減らし、効率を向上させることができます - コスト削減:メール送信のプロセスを自動化することで、人件費を削減し、他の業務にリソースを再配分することが可能になります。
- カスタマイズ性:メールの内容、形式、送信タイミングなどを完全にカスタマイズできるため、具体的な業務要件に合わせた柔軟な対応が可能です。
本記事では、Pythonでメール送信を実行するsend_mail関数を作成して処理する方法を紹介します。
※SMTPs(SMTP over SSL)でメール送信する例となります。
事前準備
サンプルコード実行用にcandy.pngとクッキー.jpgのファイルを以下からダウンロードします。
ダウンロード後は以下の図のようにCドライブにtestfileというフォルダを作成し、その中に上記2ファイルをコピーしてください。
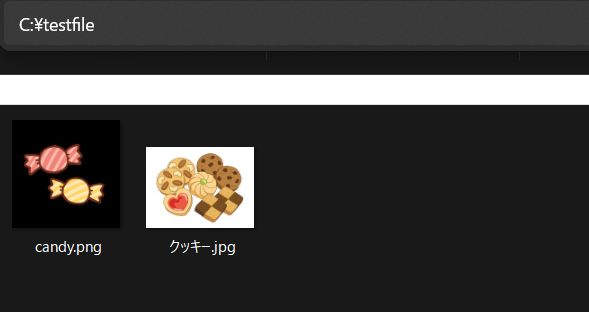
send_mail関数のサンプルコード
次に、send_mail関数のサンプルコードを紹介します。
関数の下にはテスト用のコードも記載していますので参考にしてください。
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
from email.header import Header
def send_email(
smtp_server,
smtp_port,
sender_user,
sender_password,
sender_email,
recipient_email,
subject, message,
attachment_paths = None
):
"""
メールを送信する関数。
Args:
smtp_server (str): smtpサーバ
smtp_port (str): smtpポート
sender_user (str): smtp ユーザー
sender_password= (str): smtp パスワード
sender_email (str): 送信者のemail
recipient_email (str): 受信者のemail
subject (str): 題名
message (str): 本文
attachment_paths (list, optional): 添付ファイルのパスのリスト
"""
# メールの設定
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = recipient_email
msg['Subject'] = Header(subject, 'utf-8') # 題名に日本語を含む場合の例
# メール本文
body = MIMEText(message, 'plain', 'utf-8')
msg.attach(body)
try:
# 添付ファイルが指定されている場合
if attachment_paths:
for attachment_path in attachment_paths:
attachment = MIMEApplication(open(attachment_path, 'rb').read())
attachment.add_header('Content-Disposition', 'attachment', filename=attachment_path)
msg.attach(attachment)
# SMTP over SSLでメール送信
smtp_connection = smtplib.SMTP_SSL(smtp_server, smtp_port)
smtp_connection.login(sender_user, sender_password)
smtp_connection.sendmail(sender_email, recipient_email, msg.as_string())
smtp_connection.quit()
print("メールが送信されました。")
except Exception as e:
print("メールの送信中にエラーが発生しました:", str(e))
##############################################################
# 関数のテスト
if __name__ == "__main__":
#パラメータを設定する。
smtp_server = "smtp.commufa.jp"
smtp_port = "465"
sender_user = "sender_user" #smtp ユーザー
sender_password = "sender_password" #smtp パスワード
sender_email = "aaaa@commufa.jp" #送信者 email
recipient_email = "bbbb@gmail.com" #受信者 email
subject = "お菓子"
message = "こんなお菓子が食べたいな。"
#添付ファイルがある場合にはpathを指定する。
attachment_paths = ['C:\\testfile\\candy.png', 'C:\\testfile\\クッキー.jpg']
send_email(
smtp_server,
smtp_port,
sender_user,
sender_password,
sender_email,
recipient_email,
subject,
message,
attachment_paths
)
サンプルを実行する前にsmtp_server からrecipient_email のパラメータを環境に合わせて設定してください。筆者はcommufaを利用しているので、commufaユーザーの方はsmtpサーバやポート番号はそのまま利用できると思います。
実行結果
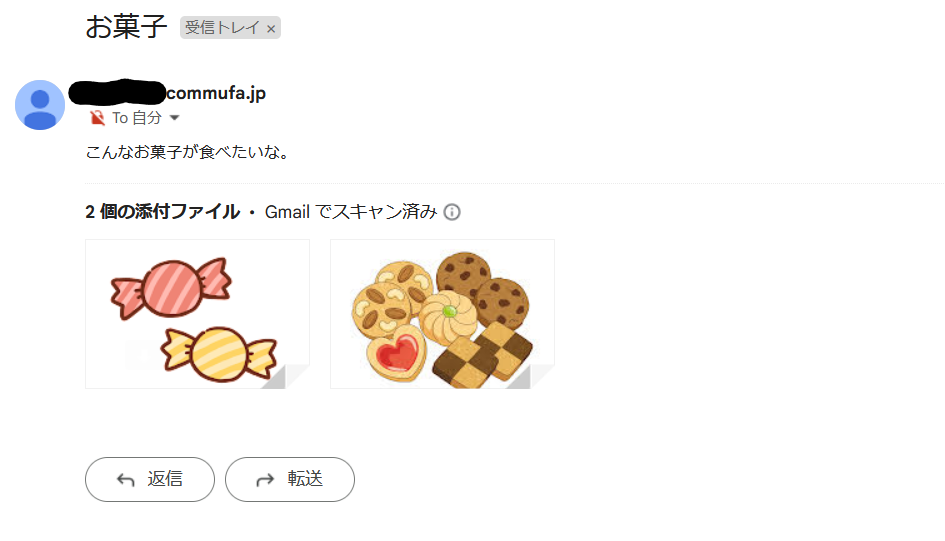
添付ファイル付きのemailが送信されます。
解説
ファイル添付しない場合
send_email(
smtp_server,
smtp_port,
sender_user,
sender_password,
sender_email,
recipient_email,
subject,
message,
# attachment_paths
)
attachment_pathsを引数で指定しなければ添付ファイルなしで処理されます。
固定パラメータについて
smtpサーバや、ポート、smtpユーザやsmtpパスワードはプログラムで書く場合は固定値がほとんどだと考えられます、この場合はiniファイル等の読み込みを行うことでソース記述を削減できますので検討してみてください。
最後に
以上がPythonでメール送信を行う方法です。
メールであればバッチ処理のエラー出力やデータ更新情報をメールソフトからスマートフォン経由で参照出来るなど日々のルーティンに組み込むことも容易ですので、利用検討してみてはいかがでしょうか。
また、メール受信の記事もありますので興味のある方は参照してみてはいかがでしょうか。
コメント